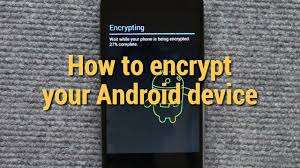
-
Contents
- 0.1 Importance of file encryption and decryption in Android
- 0.2 Overview of the topic:
- 0.3 Understanding File Encryption and Decryption
- 0.4 What is file encryption?
- 0.5 What is file decryption?
- 0.6 Importance of encryption and decryption for data security
- 0.7 Choosing an Encryption Algorithm
- 0.8 Overview of encryption algorithms available in Android
- 0.9 Selecting the appropriate encryption algorithm for your needs
- 0.10 Generating Encryption Keys
- 0.11 Generating symmetric encryption keys
- 1 Q1: What is the difference between symmetric and asymmetric encryption?
- 2 Q2: How do I choose the appropriate encryption algorithm for my Android application?
- 3 Q3: How can I securely generate encryption keys in my Android application?
- 4 Q4: What are some best practices for secure key management in Android?
- 5 Q5: How can I handle errors and exceptions in the encryption and decryption processes?
- 6 Q6: Are there any specific security considerations for transmitting and storing encryption keys in Android applications?
- 7 Q7: How can I ensure ongoing security for encrypted files in my Android application?
Importance of file encryption and decryption in Android
File encryption and decryption are crucial in maintaining data security on Android devices. Encryption transforms sensitive data into an unreadable format, ensuring confidentiality and protecting it from unauthorized access. Decryption allows authorized users to regain access to the encrypted data, providing the necessary functionality while maintaining data integrity.
-
Overview of the topic:
Encrypting and decrypting files programmatically on Android
This blog post provides a comprehensive guide on programmatically encrypting and decrypting files on Android. We will cover various aspects, including choosing the right encryption algorithm, generating encryption keys, implementing encryption and decryption processes, ensuring key security, handling errors, and following best practices for robust file security.
-
Understanding File Encryption and Decryption
-
What is file encryption?
File encryption is converting plain text or data into an unreadable format using an encryption algorithm. This ensures that unauthorized individuals cannot access or understand the data without the encryption key. Encryption algorithms, such as AES (Advanced Encryption Standard), provide strong security and are widely used for file encryption.
-
What is file decryption?
File decryption is the reverse process of encryption. It involves converting encrypted data to its original form using the correct decryption algorithm and key. Decryption allows authorized users to regain access to the encrypted data and view its contents.
-
Importance of encryption and decryption for data security
Encryption and decryption are essential for maintaining data security. Encryption protects data from unauthorized access, ensuring its confidentiality even if the device or files are compromised. Decryption allows authorized users to access and utilize the data in its original form, enabling the intended functionality without compromising security.
-
Choosing an Encryption Algorithm
-
Overview of encryption algorithms available in Android
Android provides a range of encryption algorithms that can be used for file encryption and decryption. Common algorithms include AES, DES (Data Encryption Standard), and RSA (Rivest-Shamir-Adleman). Each algorithm has strengths and considerations, such as security level, performance, and compatibility.
-
Selecting the appropriate encryption algorithm for your needs
The choice of encryption algorithm depends on various factors, including the level of security required, performance considerations, and compatibility with other systems or platforms. AES is a strong and secure encryption algorithm recommended for most use cases. However, evaluating your specific requirements and consulting relevant cryptographic guidelines or experts is important to choose the most suitable algorithm.
-
Generating Encryption Keys
-
Generating symmetric encryption keys
Symmetric encryption algorithms, such as AES, use the same key for encryption and decryption. The key needs to be securely generated and kept confidential. Android provides cryptographic libraries and functions to create secure symmetric encryption keys. Random number generators and certain key storage mechanisms, such as Android Keystore, should be utilized to ensure key security.
- Generating asymmetric encryption keys
Asymmetric encryption algorithms, like RSA, use a pair of keys: a public key for encryption and a private key for decryption. Android provides APIs for generating key pairs securely. Public keys can be shared openly, while private keys must be kept confidential and protected. When generating asymmetric encryption keys, key size, and cryptographic best practices should be considered.
- Encrypting Files Programmatically
- Reading the file to be encrypted
To programmatically encrypt a file, it is necessary to read its contents. Android provides various APIs for file handlings, such as File Input/Output streams or Content Providers, which can be used to read the file’s data.
- Encrypting the file using the chosen encryption algorithm and key
Once the file is read, it can be encrypted using the selected encryption algorithm and the generated encryption key. Android cryptographic libraries provide functions and classes for encryption operations. The file contents are processed and transformed into an encrypted format, ensuring the confidentiality and security of the data. The encrypted file can then be stored or transmitted securely.
- Storing Encrypted Files
- Choosing a secure storage location for encrypted files
Selecting a secure storage location to protect encrypted files on Android devices is crucial. The Android platform offers various options for secure file storage, such as internal storage, external storage with appropriate permissions, or encrypted databases. Consider the sensitivity of the data and the level of protection required when choosing the storage location.
- Implementing secure file storage techniques
To ensure the security of encrypted files,
additional measures can be implemented. This may include encrypting the storage location, utilizing strong file naming conventions, enforcing appropriate access controls and permissions, and regularly monitoring and updating security measures. Android security best practices, such as leveraging Android Keystore for key storage or implementing file integrity checks, should be considered for enhanced security.
- Decrypting Files Programmatically
- Retrieving the encrypted file
The encrypted file must be accessed to decrypt a file programmatically on Android. Similar to the encryption process, Android file-handling APIs can be utilized to retrieve the encrypted file’s contents.
- Decrypting the file using the appropriate decryption algorithm and key
Once the encrypted file is retrieved, it can be decrypted using the corresponding decryption algorithm and the correct decryption key. Android cryptographic libraries provide functions and classes for decryption operations. The encrypted file contents are processed, and the data is transformed to its original form, allowing authorized users to access and utilize the decrypted information.
- Ensuring Key Security
- Securely storing encryption keys in Android
The security of encryption keys is vital for maintaining the overall safety of the encryption and decryption processes. Android provides the Android Keystore system, which offers a secure and hardware-backed environment for key storage. Storing keys in the Keystore system ensures they are protected from unauthorized access and prevents key extraction.
- Implementing key protection mechanisms
In addition, key protection mechanisms should be implemented to secure key storage. This may involve utilizing key aliases, enforcing strong user authentication before accessing keys, or applying key usage policies to control key access and usage. Following Android security best practices and guidelines to safeguard encryption keys effectively is essential.
- Handling Errors and Exceptions
- Identifying and handling encryption/decryption errors
During the encryption and decryption processes, various errors or exceptions can occur. These may include incorrect key usage, file corruption, or hardware-related issues. Proper error-handling techniques should be implemented to gracefully handle such situations, ensuring that users are informed of errors and appropriate actions to mitigate the impact.
- Implementing error handling and exception management
Android provides exception-handling mechanisms, such as try-catch blocks, to handle errors effectively. It is important to log and track errors, provide meaningful error messages to users, and implement fallback strategies or recovery mechanisms where possible. Thorough testing and debugging of the encryption and decryption processes can help identify potential errors and ensure robust implementation.
- Testing and Debugging Encryption and Decryption Processes
- Creating test scenarios for encryption and decryption
Comprehensive testing should be performed to ensure the reliability and effectiveness of the encryption and decryption processes. Test scenarios should cover file types, encryption algorithms, key sizes, and potential error cases. This will help identify any issues or vulnerabilities and allow for refinement of the encryption and decryption implementations.
- Debugging and troubleshooting common issues
During testing, it is common to encounter issues or errors that require debugging and troubleshooting. Proper logging and debugging techniques and a thorough understanding of the encryption and decryption processes can help identify and resolve any issues efficiently. Addressing any identified vulnerabilities or weaknesses and refining the implementation based on the test results is important.
- Best Practices for File Encryption and Decryption in Android
- Implementing proper key management techniques
Effective key management is critical for maintaining the security of encrypted files. This includes generating keys securely, storing them in a protected environment, and implementing appropriate key rotation and revocation strategies. Adhering to cryptographic best practices and standards ensures the integrity of the encryption and decryption processes.
- Regularly updating encryption algorithms and libraries.
Cryptography is continuously evolving, and new vulnerabilities or weaknesses may be discovered in encryption algorithms over time. It is essential to stay updated with the latest security recommendations and patches the Android platform provides. Regularly updating encryption algorithms and libraries helps mitigate potential security risks and ensure the use of the most robust and secure cryptographic techniques available.
- Implementing secure coding practices
Writing secure code is crucial for ensuring the integrity of the encryption and decryption processes. Safe coding practices, such as input validation, memory handling, and secure communication protocols, helps mitigate potential vulnerabilities, such as buffer overflows or side-channel attacks. Code reviews, static analysis tools, and security testing should be employed to identify and address any security flaws in the implementation.
- Protecting against brute-force attacks
To protect against brute-force attacks, where an attacker attempts to guess the encryption key by systematically trying all possible combinations, it is important to enforce strong password policies and consider using key-strengthening techniques, such as key derivation or password-based key derivation functions. This makes it computationally expensive and time-consuming for an attacker to guess the key.
- Ensuring secure transmission and storage of keys
When transmitting or storing encryption keys, using secure channels and protocols is crucial. Key exchange protocols, such as the Diffie-Hellman key exchange, can be utilized to establish certain communication channels between devices. Additionally, secure storage mechanisms, such as Android Keystore or hardware security modules, should be leveraged to protect keys from unauthorized access or extraction.
- Regularly auditing and monitoring encryption processes.
Regular auditing and monitoring of the encryption and decryption processes help identify suspicious activities, unauthorized access attempts, or potential vulnerabilities. Implementing logging and monitoring mechanisms and proper security event management allows for timely detection and response to security incidents, ensuring the ongoing integrity and security of the encrypted files.
- Educating users on data security best practices
Users play a crucial role in data security. Educating users on best practices, such as using strong passwords, protecting their devices with secure screen locks, and being cautious about sharing sensitive information, enhances the overall security of the encrypted files. User awareness and responsible data handling contribute to a robust security posture.
In conclusion, programmatically encrypting and decrypting files in Android involves:
- Understanding encryption algorithms.
- Generating secure encryption keys.
- Implementing the encryption and decryption processes.
- Ensuring key security.
- Handling errors.
- Following best practices.
By incorporating strong encryption techniques, secure key management, and robust security measures, developers can protect sensitive data on Android devices and maintain data integrity and confidentiality. Adhering to security guidelines and regularly updating cryptographic implementations ensures a proactive approach to data security in Android applications.
Q1: What is the difference between symmetric and asymmetric encryption?
A1: Symmetric encryption uses a single key for encryption and decryption processes. The same key is used to both scramble and unscramble the data. In contrast, asymmetric encryption uses a pair of keys: a public key for encryption and a private key for decryption. The public key can be freely shared, while the private key must be kept secure. Symmetric encryption is generally faster and more efficient for encrypting large amounts of data, while asymmetric encryption provides enhanced security for tasks such as secure key exchange and digital signatures.
Q2: How do I choose the appropriate encryption algorithm for my Android application?
A2: The choice of encryption algorithm depends on various factors, including the level of security required, performance considerations, and compatibility with other systems or platforms. The Advanced Encryption Standard (AES) is recommended for most scenarios. AES supports different key sizes and is widely regarded as secure and efficient. When selecting an algorithm, consider factors such as key length, resistance against known attacks, and the recommendations provided by cryptographic experts and standards organizations.
Q3: How can I securely generate encryption keys in my Android application?
A3: Secure key generation is crucial for maintaining the overall security of encryption processes. Android provides cryptographic libraries and functions that offer secure random number generation for key generation. The `java.security.SecureRandom` class can generate cryptographically secure random numbers. The Android Keystore system also provides a secure environment for key generation and storage, leveraging hardware-backed security features for enhanced protection against key extraction.
Q4: What are some best practices for secure key management in Android?
A4: Secure key management protects encryption keys from unauthorized access and ensures their confidentiality and integrity. Some best practices include storing keys in secure environments like the Android Keystore system, using key aliases for improved security, enforcing proper access controls, and implementing key rotation and revocation strategies.
It is crucial to follow cryptographic guidelines and recommendations, regularly update keys when necessary, and leverage hardware-backed security features to enhance key protection.
Q5: How can I handle errors and exceptions in the encryption and decryption processes?
A5: Proper error handling and exception management are essential to ensure the reliability and integrity of encryption and decryption processes. Exception-handling mechanisms, such as try-catch blocks, should be implemented to handle errors and exceptions gracefully.
It is important to provide meaningful error messages to users, log and track errors for debugging purposes, and implement fallback strategies or recovery mechanisms where applicable. Thorough testing and validation can help identify and address potential errors and vulnerabilities.
Q6: Are there any specific security considerations for transmitting and storing encryption keys in Android applications?
A6: Transmitting and storing encryption keys require special attention to ensure security. When transmitting keys, use secure communication channels and protocols, such as HTTPS or secure socket layers (SSL/TLS). Key exchange protocols, like the Diffie-Hellman key exchange, can establish certain channels between devices. To protect keys from unauthorized access or extraction, leverage secure storage mechanisms provided by Android, such as the Android Keystore system or hardware security modules.
Q7: How can I ensure ongoing security for encrypted files in my Android application?
A7: Regular auditing and monitoring can ensure ongoing security for encrypted files. Implement logging and monitoring mechanisms to detect suspicious activities or unauthorized access attempts.
Regularly audit the encryption and decryption processes, review security event logs, and update cryptographic implementations to address any vulnerabilities or weaknesses that may be discovered. Educating users about data security best practices and promoting responsible data handling also contributes to the ongoing security of encrypted files.